03 Apr 2024
The world of Decentralized Finance (DeFi) offers exciting new opportunities for cryptocurrency users. But how does it all work? A key concept in DeFi is the liquidity pool, and platforms like Aave are built around them. Let’s dive in and explore what liquidity pools are, how Aave utilizes them, and the benefits and drawbacks of this innovative platform.
Understanding Liquidity Pools
Imagine a giant pot of crypto assets. Anyone can add funds (deposit), and anyone can borrow from this pot (withdraw). This pot is essentially a liquidity pool. They fuel DeFi by providing the necessary assets for lending and borrowing activities. Users who deposit their crypto into the pool earn interest on their holdings, while borrowers can access funds at market-determined rates.
Aave: A Liquidity Powerhouse
Aave is a prominent DeFi platform that leverages liquidity pools. Here’s how it works:
- Supplying Crypto: Users can deposit various cryptocurrencies into Aave’s liquidity pools. These deposits earn interest as borrowers pay back their loans.
- Borrowing Crypto: Users can borrow crypto from the pool by putting up collateral (another cryptocurrency). The interest rate on these loans depends on various factors like supply and demand for the specific crypto.
- Earning AAVE: Aave has its own governance token, AAVE. By holding AAVE, users can participate in the platform’s governance and potentially earn additional rewards.
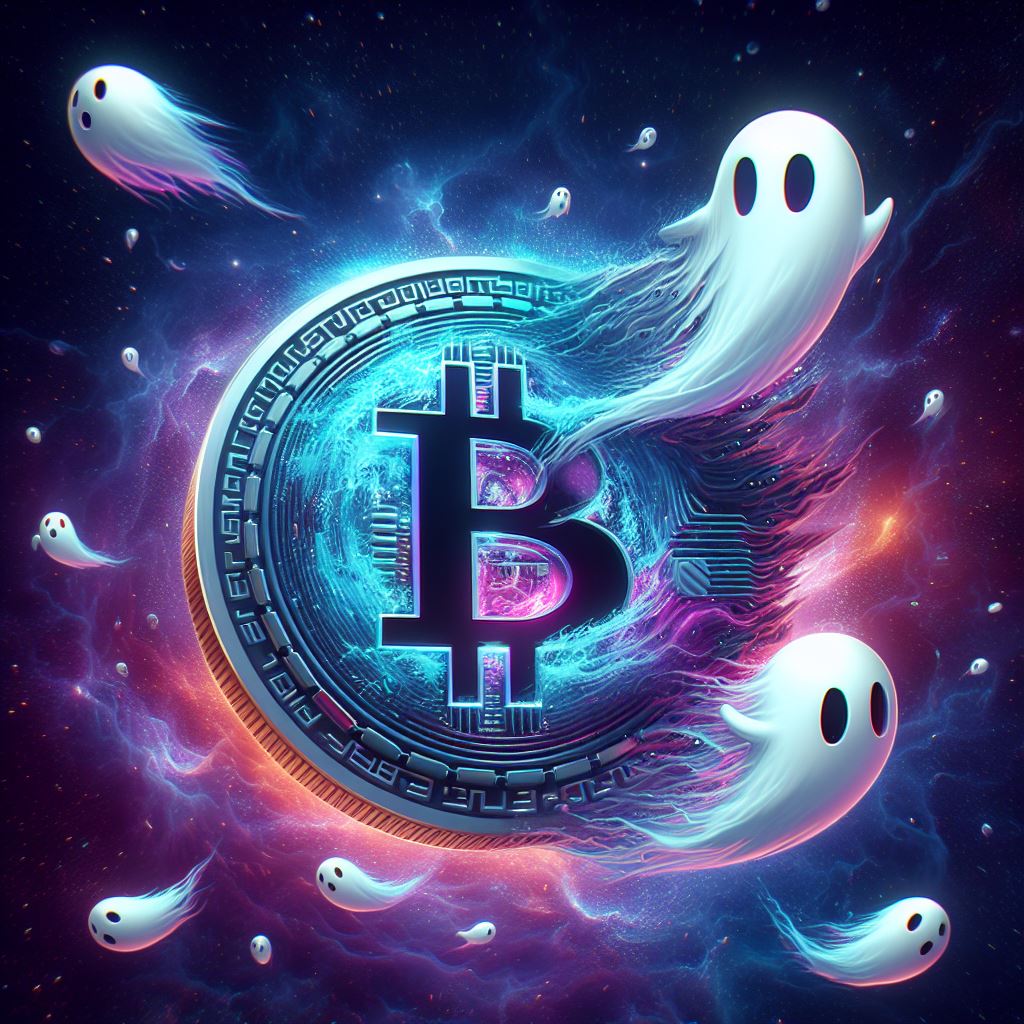
Benefits of Aave
- Passive Income: Supplying crypto to Aave’s pools offers a way to earn interest on your holdings without actively trading.
- Flexible Borrowing: Borrowers can access various cryptocurrencies at competitive rates.
- Transparency & Security: Aave is an open-source platform, meaning its code is publicly available for scrutiny.
Additionally, smart contracts automate transactions, reducing the risk of human error or manipulation.
Drawbacks to Consider
- Volatility: Cryptocurrency prices can fluctuate significantly. Supplying crypto to liquidity pools exposes you to potential impermanent loss, where the value of your deposit might be lower when you withdraw compared to simply holding the asset.
- Smart Contract Risk: Smart contracts, while generally secure, can have vulnerabilities. A successful exploit could lead to loss of funds deposited in the pool.
- Complexity: DeFi can be complex for beginners. Understanding interest rates, collateralization ratios, and potential risks is crucial before diving in.
Conclusion
Liquidity pools are the backbone of DeFi, and Aave is a leading platform that utilizes them effectively. While Aave offers attractive opportunities for earning interest and borrowing crypto, it’s important to be aware of the potential risks involved. As with any investment, careful research and understanding the underlying technology are crucial before participating in DeFi.
18 Mar 2024
Introduction to Meme Coins
Meme coins, as highlighted in the article from Investopedia are a unique category of cryptocurrencies that have gained popularity through social media trends and viral marketing. Unlike traditional cryptocurrencies driven by technological advancements or real-world utility, meme coins derive their value primarily from community involvement and social media excitement. These digital assets have become a cultural phenomenon, offering emotional support or recognition to specific communities despite lacking substantial economic value.
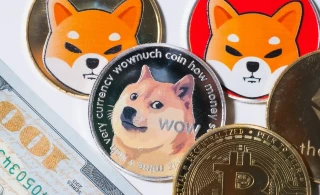
Why Meme Coins Lack Utility
While meme coins can provide emotional backing to certain groups, they are often criticized for their lack of real-world application and limited utility. These coins are primarily driven by hype and social media trends rather than fundamental value or technological innovation. Critics argue that meme coins do not contribute significantly to the cryptocurrency ecosystem in terms of solving real-world problems or advancing blockchain technology.
Criticizing the Meme Coin Frenzy
Vitalik Buterin’s critique of meme coins, sheds light on the investment frenzy surrounding these digital assets. Buterin emphasizes the need for more meaningful engagement and support within the crypto infrastructure, highlighting the importance of balancing investment to foster a more equitable and functional ecosystem. The criticism points towards the speculative nature of meme coins and the risks associated with investing in assets driven primarily by social media influence rather than intrinsic value.
In conclusion, while meme coins offer a unique avenue for high-risk, high-reward investments and have captured significant attention in the cryptocurrency landscape, it is essential for investors to approach them with caution. Understanding the limitations of meme coins in terms of utility and being aware of the potential risks associated with market speculation is crucial for making informed investment decisions in this dynamic sector. Conducting thorough research, staying updated on market trends, and diversifying investments are key strategies to navigate the meme coin market responsibly.
29 Feb 2024
In recent developments within the Ethereum community, Vitalik Buterin, the co-founder of Ethereum, has proposed an innovative approach to bolstering security in blockchain projects. Buterin’s idea revolves around leveraging artificial intelligence (AI) for code audits, aiming to reduce bugs and enhance the overall integrity of Ethereum projects.
Vitalik Buterin’s Vision for AI-Assisted Code Audits
Vitalik Buterin’s proposal suggests integrating AI into the code auditing process to improve efficiency and effectiveness. By harnessing AI capabilities, developers can enhance their ability to detect vulnerabilities and ensure the robustness of smart contracts within the Ethereum ecosystem
The Role of AI in Smart Contract Audits
AI-assisted code audits offer a unique advantage over traditional automated tools by adapting and learning from new information. This adaptability is crucial in the dynamic landscape of smart contract security, where emerging vulnerabilities require swift detection and mitigation strategies
Industry Response and Potential Benefits
Ethereum project developers have shown support for Buterin’s idea, emphasizing the potential of combining human expertise with AI systems to create a comprehensive security framework. The collaboration between AI and human inspection could significantly strengthen code integrity and mitigate risks associated with bugs and exploits
Addressing Security Challenges in Blockchain Projects
The increasing complexity of blockchain projects necessitates advanced security measures to safeguard user funds and maintain trust within the ecosystem. By exploring AI-based solutions for code audits, Ethereum aims to proactively address security challenges and enhance the resilience of its decentralized applications
In conclusion, Vitalik Buterin’s proposal to incorporate AI into code audits represents a significant step towards fortifying Ethereum’s security infrastructure. By embracing innovative technologies like AI, Ethereum demonstrates its commitment to advancing blockchain security standards and fostering a more secure environment for decentralized applications.
26 Jan 2024
Jetpack Compose has introduced a modern way of building user interfaces for Android applications, and part of its appeal lies in its robust testing capabilities. Let’s delve into the world of Jetpack Compose UI testing in Android, exploring insights from various sources to understand the importance and implementation of testing in Jetpack Compose.
Easy Setup
Jetpack Compose provides a streamlined approach to setting up UI tests. By adding the necessary dependencies to the build.gradle
file, developers can easily incorporate Compose testing libraries into their projects. This simplified setup ensures that developers can focus on writing effective tests without the hassle of complex configurations.
Enhanced Test Implementation
Jetpack Compose UI testing allows developers to write tests that focus on specific UI components and interactions. By following best practices and structuring tests around states and events, developers can ensure that their UI composable functions are thoroughly tested. This approach enhances the reliability and robustness of UI components, leading to a more stable and user-friendly application.
Comprehensive Test Rule Integration
Jetpack Compose offers test rule integration that simplifies the process of writing and executing UI tests. Developers can leverage test rules like createComposeRule() or createAndroidComposeRule() to set up test environments and interact with UI components effectively. These test rules provide a structured framework for writing test functions and validating UI behavior.
Real-time Testing Feedback
Jetpack Compose UI testing provides real-time feedback on UI interactions, allowing developers to validate changes and ensure that the app behaves as expected. By utilizing features like assertExists(), performClick(), and assertDoesNotExist(), developers can simulate user interactions and verify the responsiveness of their UI components. This real-time feedback loop accelerates the testing process and aids in identifying potential issues early on.
Test Tag Implementation
Jetpack Compose introduces the concept of test tags, which serve as references for making specific components detectable in tests. By assigning test tags to composable functions, developers can easily locate and interact with UI elements during testing. This approach enhances test readability and maintainability, making it easier to identify and troubleshoot issues within the UI.
Don’t overuse testTag once it may pollute your production code. Instead of that, you can use semantics to interact with the UI on your tests. Using semanatics improves your app’s accessibility once Android utilizes semantics to offer alternative interaction methods for users with special needs, such as TalkBack, a screen reader, or Switch Access.
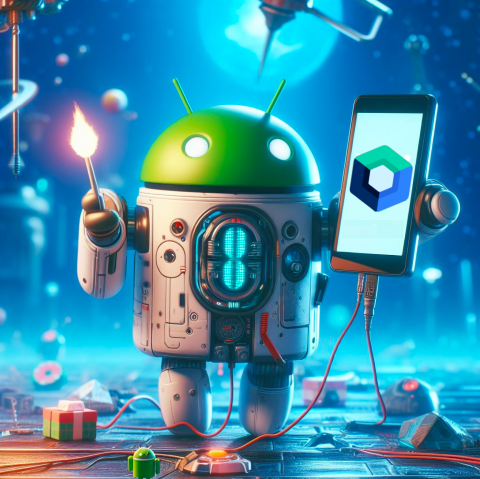
In conclusion, Jetpack Compose UI testing in Android offers a comprehensive framework for validating UI components, interactions, and behaviors. By leveraging the testing capabilities provided by Jetpack Compose, developers can ensure the quality, reliability, and performance of their Android applications, leading to enhanced user experiences and increased app stability.
15 Nov 2023
Android Jetpack Compose stands out as a powerful toolkit that empowers developers to build modern and engaging user interfaces for Android applications. Let’s continue exploring the benefits of Jetpack Compose based on the insights gathered from various sources.
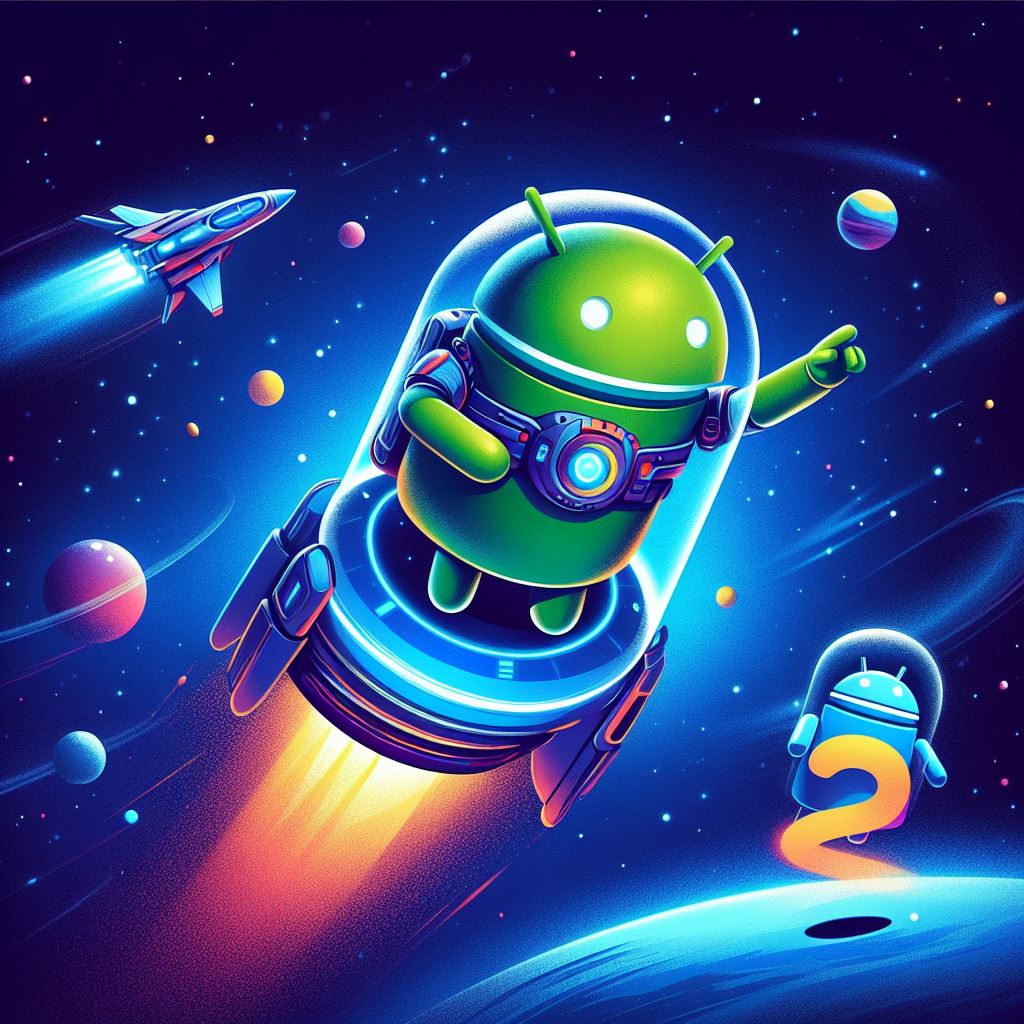
Seamless Integration with Existing Code
Jetpack Compose is designed to be compatible with existing codebases, allowing developers to adopt it gradually and integrate it seamlessly into their projects. This compatibility ensures that developers can leverage the power of Compose without the need for a complete overhaul of their existing codebase, enabling a smooth transition to modern UI development practices.
Live Previews and Full Android Studio Support
Jetpack Compose accelerates development by providing live previews and full support within Android Studio. Developers can visualize changes to the UI in real-time, iterate quickly, and ensure a consistent user experience across different screen sizes and orientations. The robust tooling support offered by Compose enhances developer productivity and facilitates a more efficient development workflow.
Jetpack Compose offers direct access to Android platform APIs, enabling developers to create feature-rich and visually appealing apps with ease. By leveraging built-in support for Material Design, Dark theme, animations, and more, developers can create apps that adhere to Android design guidelines and provide a seamless user experience.
Adaptive UI Development
Compose for large screens and Wear OS extends the capabilities of Jetpack Compose to cater to different form factors and screen sizes. The adaptive layout ensures that the UI of the app responds effectively to varying screen dimensions, orientations, and device types, providing a consistent and optimized user experience across all platforms.
In conclusion, Android Jetpack Compose emerges as a game-changer in the realm of Android UI development, offering a plethora of benefits that enhance developer productivity, streamline UI creation, and elevate the user experience of Android applications. By embracing Jetpack Compose, developers can unlock new possibilities in UI design and development, leading to the creation of visually stunning and feature-rich Android apps.