12 Feb 2023
Jetpack Compose is a modern UI toolkit by Google that simplifies and accelerates Android app development. With its declarative approach, Jetpack Compose allows developers to build beautiful and responsive user interfaces with less code. For developers who have been working with XML-based UI in Android, migrating to Jetpack Compose might seem like a daunting task. However, with some understanding of the process and best practices, the migration can be smooth and rewarding. In this blog post, we will explore the process of migrating existing XML-based UI code to Jetpack Compose, along with some best practices and common challenges.
Understanding the Basics
Before diving into the migration process, it’s important to understand the basics of Jetpack Compose. Jetpack Compose uses a completely different approach to building UI compared to XML-based UI. Instead of defining UI elements in XML files, Jetpack Compose uses composable functions to create the user interface. Composable functions are lightweight and modular, allowing for easier composition and reuse. With Jetpack Compose, UI elements are defined using simple Kotlin functions that return UI components, making the code more concise and readable.
Step-by-Step Migration Process
- Identify the Scope of Migration: Start by determining the scope of the migration. Analyze your XML-based UI code and identify the screens or components that you want to migrate to Jetpack Compose. It’s a good idea to start with smaller, less complex screens for the initial migration.
- Create a Composable Function: For each screen or component you want to migrate, start by creating a new composable function in your Jetpack Compose code. This function will replace the XML file and will be responsible for defining the UI elements and layout.
- Translate XML Elements to Compose: Analyze the XML code and translate each XML element and its attributes to the equivalent Jetpack Compose code. For example, if you have a TextView in XML, you would replace it with a Text composable function in Jetpack Compose.
- Refactor Layouts: XML-based layouts often use LinearLayout, RelativeLayout, or ConstraintLayout for positioning UI elements. In Jetpack Compose, you can use the powerful layout composable functions like Column, Row, and Box to achieve the desired layout. Refactor your layout code accordingly.
- Handle Styling: XML-based UI often relies on styles and themes for consistent styling. In Jetpack Compose, you can use the Material Design Components library to apply styles and themes. Refactor your styling code to use the appropriate Compose functions and APIs.
- Migrate Business Logic: Along with the UI code, you’ll also need to migrate any associated business logic. This could include event handling, data binding, or any other logic related to the UI. Update your code to use the Jetpack Compose equivalents, such as using onClick instead of onClickListener for handling click events.
- Gradual Migration: Consider adopting a gradual migration approach. Instead of migrating all screens at once, you can start by migrating individual screens or components and gradually expand the migration. This allows you to validate the changes, adapt to the new workflow, and measure the impact before fully migrating your entire application.
Best Practices
Here are some best practices to keep in mind during the migration process:
- Start with Small Screens - Begin the migration process with smaller, less complex screens or components. This will help you learn and understand the Compose syntax and concepts before moving on to more complex parts of your application.
- Refactor Incrementally - Instead of rewriting your entire XML-based UI codebase, refactor and migrate sections incrementally. This ensures that your app remains functional during the migration and reduces the chances of introducing bugs.
- Leverage Compatibility Libraries - Jetpack Compose provides compatibility libraries that allow you to use Compose alongside your existing XML-based UI code. You can gradually start using Compose in existing activities or fragments before fully migrating them.
- Validate and Test - Thoroughly test and validate your migrated screens or components to ensure they are visually and functionally equivalent to the XML-based UI. Writing unit tests and UI tests for your Compose code is crucial to catch any potential issues.
Common Challenges
Migrating from XML-based UI to Jetpack Compose can come with its fair share of challenges. Here are a few common challenges you might encounter:
- Learning Curve - Jetpack Compose introduces a new syntax and concept. It takes time to get familiar with the Compose way of building UI, especially if you are coming from an XML-based UI background.
- Interoperability with Existing Code - Integrating Jetpack Compose with existing XML-based UI code can be challenging. You might need to find workarounds or use compatibility libraries to bridge the gap between the two approaches.
- Integrating with 3rd Party Libraries - Some 3rd party libraries might not have built-in support for Jetpack Compose. You might need to find alternative libraries or write custom wrappers to integrate them seamlessly.
- Limited Documentation and Resources - Since Jetpack Compose is still relatively new, you might find limited documentation and resources compared to XML-based UI. However, the Jetpack Compose documentation and community are continuously growing.
Jetpack Compose official documentation
Migrating an app to use Jetpack Compose
Material Design Components for Jetpack Compose
Jetpack Compose Samples GitHub repository
16 Jan 2023
sendTransaction
and eth_sendPrivateTransaction
are both methods for sending transactions on the Ethereum blockchain, but there are some significant differences between them.
sendTransaction
is a method that is used to send transactions on the public Ethereum network. It is a standard method that is available in all Ethereum clients and is used to send transactions from one public account to another public account. The transaction details, such as sender address, recipient address, transaction amount, gas price, and gas limit, are publicly visible on the blockchain.
On the other hand, eth_sendPrivateTransaction
is a method that is used to send private transactions on a private Ethereum network. Private networks are typically used in enterprise settings where transactions need to be kept confidential. The transaction details, including the smart contract code, are visible only to the parties involved in the transaction. This is achieved using private contracts and private transactions that are only visible to a subset of nodes on the private Ethereum network.
eth_sendPrivateTransaction
is relevant because it provides a way for enterprises to use the Ethereum blockchain to conduct business in a private and secure manner. By keeping transactions confidential, companies can protect their proprietary information and maintain the privacy of their customers. Additionally, private Ethereum networks can provide faster transaction times and lower transaction costs than the public Ethereum network.
Private Ethereum networks are important because they allow organizations to take advantage of the benefits of blockchain technology while tailoring the network to their specific needs. By offering greater privacy, customization, control, scalability, and cost-effectiveness, private Ethereum networks are an excellent choice for businesses looking to leverage blockchain technology to achieve their strategic goals.
26 Dec 2022
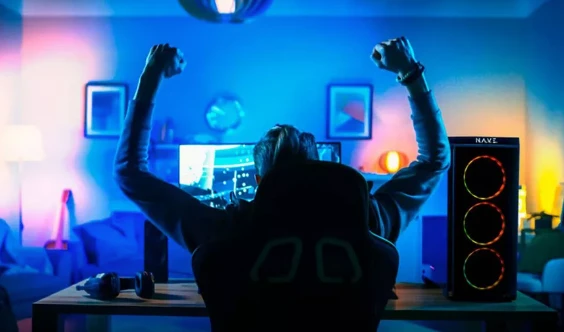
The future of gaming in the world of cryptocurrency is an exciting and rapidly evolving space. As the use of cryptocurrency and blockchain technology continues to grow and gain mainstream acceptance, it’s likely that we’ll see more and more game developers integrating these technologies into their products.
One potential use for cryptocurrency in gaming is as a means of in-game currency. In many games, players can earn or purchase virtual currency that can be used to buy in-game items or unlock new content. By using cryptocurrency as the medium of exchange, players could potentially buy and sell these virtual items on external marketplaces, potentially even making real-world profits from their in-game activities.
Another possibility is the use of cryptocurrency and blockchain technology to enable cross-game item interoperability. Currently, items and currency earned in one game are often not transferable to other games. By using blockchain technology to create a shared, decentralized ledger of in-game items, players could potentially take their hard-earned virtual items with them from game to game, creating a more immersive and persistent gaming experience.
In addition to these potential uses, we could also see the emergence of entirely new types of games built on blockchain technology. For example, some developers are already working on creating blockchain-based games that use non-fungible tokens (NFTs) to represent unique in-game items or assets. These NFTs could potentially be bought, sold, and traded on external marketplaces, adding a whole new level of value and ownership to in-game items.
15 Nov 2022
Kotlin is a modern, statically typed programming language that runs on the Java Virtual Machine and can also be compiled to JavaScript. It is expressive, concise, and powerful, and has quickly become a popular choice for Android development. In this blog post, we will take a look at some of the advanced features of Kotlin that can make your code more concise, readable, and efficient.
Type Inference
Type inference is a feature that allows the Kotlin compiler to infer the type of a variable or expression based on the context in which it is used. This means that you don’t always have to specify the type explicitly, and the compiler will fill it in for you.
For example, consider the following code:
val x = 1
val y = 2
val z = x + y
In this code, the variables x, y, and z are all inferred to be of type Int, because they are all assigned integer values. This is convenient because it saves you from having to specify the type explicitly, and it also makes the code more readable because it is less cluttered with type annotations.
Type inference can also be used with generic types. For example, consider the following code:
val list = listOf(1, 2, 3)
Here, the type of the list variable is inferred to be List, because it is assigned a list of integers.
Inline Functions
In Kotlin, you can use the inline keyword to annotate a function as inline. This means that the function will be inlined at the call site, which can improve the performance of your code by avoiding the overhead of function calls.
For example, consider the following code:
inline fun foo(block: () -> Unit) {
println("Before")
block()
println("After")
}
foo {
println("Inside")
}
In this code, the foo function is annotated as inline, which means that the code inside the function will be inlined at the call site. The output of this code will be:
Inlining functions can be especially useful when working with higher-order functions, which we will discuss next.
Higher-Order Functions
A higher-order function is a function that takes one or more functions as arguments or returns a function as a result. Kotlin has excellent support for higher-order functions, which can be used to write concise and expressive code.
For example, consider the following code:
fun foo(list: List<Int>, predicate: (Int) -> Boolean): List<Int> {
return list.filter(predicate)
}
val result = foo(listOf(1, 2, 3, 4, 5), { it % 2 == 0 })
In this code, the foo function is a higher-order function because it takes a function (predicate) as an argument. The predicate function is a lambda expression that takes an Int and returns a Boolean. The foo function uses the filter higher-order function to filter the list based on the predicate.
Higher-order functions can be used in combination with inline functions to write even more concise and expressive code. For example, consider the following code:
inline fun <T> Iterable<T
25 Aug 2022
If you’re using Jetpack Compose in your Android app development, you’ve likely encountered the concept of “collections”. In this blog post, we’ll explore what collections are in Jetpack Compose, how to work with them, and some best practices for using them effectively.
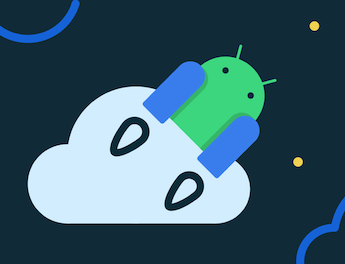
First, let’s define what collections are in Jetpack Compose. Collections are simply groups of data that can be iterated over and manipulated. This can include lists, sets, and maps. In Jetpack Compose, you can use collections to build UI elements that display multiple items, such as lists or grids.
One of the most useful ways to work with collections in Jetpack Compose is through the use of the @Composable function For. This function allows you to iterate over a collection and build a UI element for each item in the collection. For example, you could use For to build a list of items like this:
@Composable
fun MyList(items: List<Item>) {
Column {
For(items) { item ->
Text(text = item.name)
}
}
}
This will create a Text
element for each item in the items list, displaying the name property of each Item.
There are a few best practices to keep in mind when using collections in Jetpack Compose. Firstly, try to minimize the number of times you iterate over a collection. This can help improve the performance of your app, as each iteration can take time. Secondly, be mindful of the size of your collections. Large collections can lead to slower rendering times, so try to keep your collections as small as possible.
In summary, collections in Jetpack Compose are groups of data that can be iterated over and manipulated to build UI elements. The For function is a useful tool for working with collections, and it’s important to keep performance in mind when using them. By following these best practices, you can effectively use collections in your Jetpack Compose projects to build dynamic and efficient UI elements.