12 Jun 2022
Supply chain management is a critical function in any business, as it involves the procurement, production, and distribution of goods and services. It is a complex process that requires coordination and collaboration between multiple stakeholders, including suppliers, manufacturers, distributors, and customers.
Blockchain technology has the potential to revolutionize supply chain management by providing a decentralized, transparent, and secure platform for managing and tracking the flow of goods and services. Here are some key ways that blockchain can improve supply chain management:
Increased transparency: Blockchain allows for the creation of a shared, immutable record of transactions that can be accessed by all stakeholders in the supply chain. This increased transparency can help to reduce fraud and errors, and improve trust and collaboration between partners.
Improved traceability: With blockchain, it is possible to track the movement of goods from the point of origin to the final destination. This can help to improve the efficiency and accuracy of the supply chain, and reduce the risk of counterfeiting or other types of fraud.
Enhanced security: Blockchain provides a secure and decentralized platform for storing and sharing data, making it difficult for hackers to access or alter information. This can help to protect sensitive data and reduce the risk of data breaches.
Improved efficiency: By automating many of the processes involved in supply chain management, blockchain technology can help to streamline operations and reduce the need for manual intervention. This can lead to cost savings and improved efficiency.
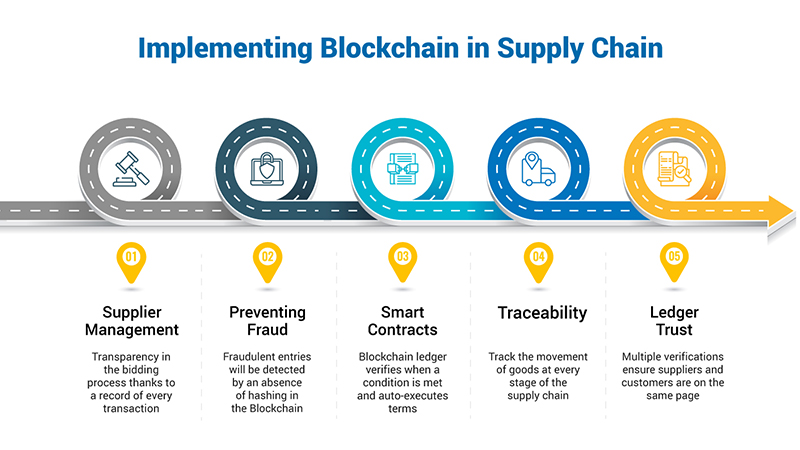
In conclusion, blockchain technology has the potential to significantly improve supply chain management by increasing transparency, improving traceability, enhancing security, and improving efficiency. As more and more businesses adopt blockchain technology, it is likely that we will see a transformation in the way supply chains are managed and operated.
27 May 2022
As Ethereum continues to grow and mature, it will be important to continue to refine and improve its governance model in order to ensure that it remains a decentralized and transparent platform that serves the needs of its users. This will require ongoing collaboration and dialogue between all stakeholders, including developers, users, and investors, to ensure that the platform remains aligned with the values and goals of the Ethereum community.
One of the main challenges facing Ethereum governance is balancing the needs of the different stakeholders involved. This includes developers, users, investors, and the Ethereum Foundation itself. Ensuring that the needs of all of these groups are taken into account can be difficult, and it requires ongoing communication and collaboration to ensure that the platform is able to meet the needs of all of these groups.
Another challenge is ensuring that the Ethereum governance process is transparent and accountable. This is especially important given the decentralized nature of the platform, as it is critical that all stakeholders have the ability to have their voices heard and their concerns addressed.
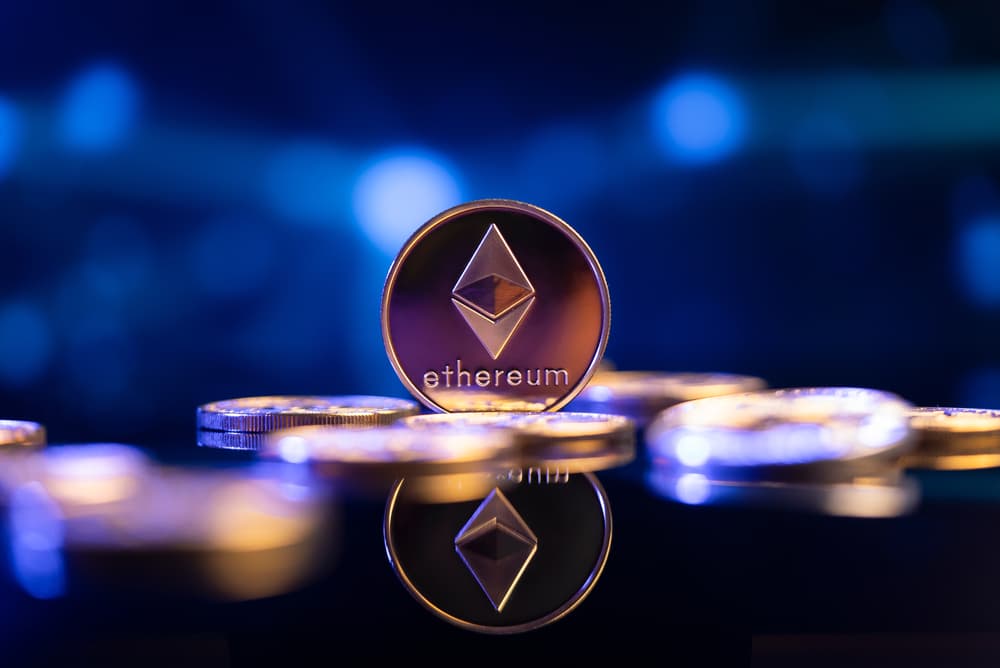
One way that Ethereum is addressing these challenges is through the use of voting mechanisms, such as the ECF, which allow for more democratic decision-making processes. In addition, the Ethereum Foundation has implemented a number of transparency initiatives, such as open meetings and the creation of a transparency portal, to ensure that stakeholders are able to stay informed about the latest developments and decisions being made within the Ethereum community.
Despite these challenges, Ethereum governance has been largely successful in ensuring that the platform is able to adapt and evolve over time. It has been able to successfully navigate significant challenges, such as the DAO hack, and has continued to grow and thrive as a result.
22 Apr 2022
As Ethereum continues to gain popularity as a platform for decentralized applications (dApps), it is important for developers to adhere to best practices and utilize design patterns in order to create robust and secure dApps.
One key best practice for Ethereum development is to use a modular architecture. This involves breaking down the dApp into smaller, more manageable components that can be tested and developed independently. This not only makes the development process more efficient, but it also makes it easier to identify and fix issues.
Another important best practice is to use version control systems such as Git to track changes to the dApp’s codebase. This allows developers to easily revert to previous versions if necessary and collaborate with other team members.
Design patterns are also essential for Ethereum development. One commonly used pattern is the factory pattern, which allows developers to create new instances of a contract without having to hardcode its address. This is useful for deploying contracts to different networks or for testing purposes.
Another useful design pattern is the upgradable contract pattern, which allows developers to make changes to a contract without having to deploy a new version. This is particularly useful for bug fixes or feature updates, as it avoids the need to migrate users to a new contract.
Overall, adhering to best practices and utilizing design patterns can help ensure the success of an Ethereum dApp. By following these guidelines, developers can create more efficient and secure dApps that are better able to meet the needs of users.
12 Mar 2022
Consensus mechanisms are an essential component of any decentralized system or blockchain. They are the process by which a group of participants in a network reach agreement on a single version of the truth. In a decentralized system, there is no central authority to enforce rules or make decisions, so the consensus mechanism is what ensures that the network operates smoothly and fairly.
There are several different types of consensus mechanisms, each with its own strengths and weaknesses. Here are some of the most common ones:
Proof of Work (PoW)
Proof of Work is the consensus mechanism that is used by the Bitcoin blockchain. It works by requiring participants (miners) to solve complex mathematical puzzles in order to validate transactions and add them to the blockchain. The first miner to solve the puzzle gets to add the block to the chain and is rewarded with a small amount of cryptocurrency.
Proof of Stake (PoS)
Proof of Stake is a consensus mechanism that uses a different approach to validate transactions. Instead of requiring miners to solve complex puzzles, it relies on a group of validators who are chosen based on the amount of cryptocurrency they hold (their stake). The more cryptocurrency a validator has, the more likely they are to be chosen to validate a transaction. This system is less resource-intensive than Proof of Work, but it has its own set of potential problems, such as the potential for validators with a large stake to dominate the process.
Delegated Proof of Stake (DPoS)
Delegated Proof of Stake is a variation of Proof of Stake that involves a group of elected representatives who are responsible for validating transactions. The representatives are chosen by the network participants through a voting process. This system is meant to be more democratic and efficient than Proof of Work, but it has faced criticism for being susceptible to centralization and bribery.
Federated Byzantine Agreement (FBA)
Federated Byzantine Agreement is a consensus mechanism that is used by the Stellar blockchain. It works by having a group of trusted nodes (called validators) that are responsible for verifying transactions. The validators reach consensus by communicating with each other and agreeing on the validity of the transaction. This system is faster and more efficient than Proof of Work, but it relies on the trustworthiness of the validators.
Proof of Authority (PoA)
Proof of Authority is a consensus mechanism that is used by private blockchains or those that require a high level of security. It works by having a group of trusted individuals or organizations (called validators) that are responsible for verifying transactions. This system is more efficient and secure than Proof of Work, but it relies on the trustworthiness and integrity of the validators.
Consensus mechanisms are an important part of any decentralized system, as they ensure that the network is secure, efficient, and fair. It’s important to carefully consider the pros and cons of different consensus mechanisms before choosing one for your project.
28 Jun 2021
Chainlink
Chainlink is a decentralized network of nodes that provide data and
information from off-blockchain sources to on-blockchain smart
contracts via oracles
Chainlink greatly expands the capabilities of smart contracts by enabling access to real-world data and off-chain computation while maintaining the security and reliability guarantees inherent to blockchain technology.
This tutorial will be based on this repo and we will use Truffle – a development environment, testing framework and asset pipeline for Ethereum
Prerequisite
1. Install NodeJS
2. Install Truffle: npm install -g truffle
3. Install Metamask
4. Install Ganache
5. Install VSCode
6. Install Solidity Plugin
Hands on: Truffle + Chainlink
npm install --global yarn
mkdir Chainlink
cd Chainlink
truffle unbox smartcontractkit/box
yarn
Running tests
Run Ganache or enable/integrate
Kovan testnet
npm test
All tests of /test
will be executed.
There are tests about creating requests with and without Link tokens, sending these requests to oracle contract addresses, and testing contract ownership.
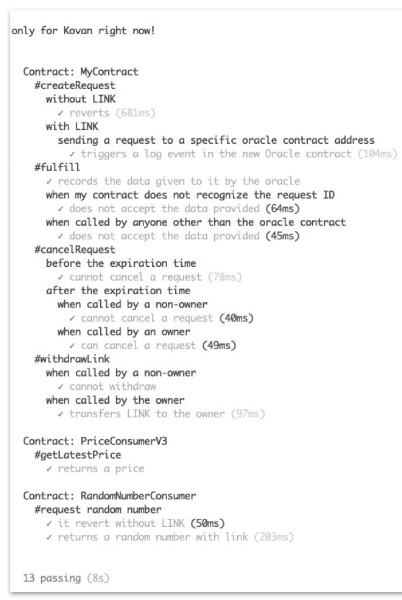
Note
You must acquire some LINK
for interact with you smart contract.
Get some LINK
via Chainlink Kovan Faucet website 🤑 🤑 🤑
Ganache deployment
truffle migrate --network ganache --reset
Kovan deployment
- Setup Metamask and connect to Kovan network
- Faucet: faucet.kovan.network
- Create account and a project using Infura.io – step-by-step tutorial
- Infura setup and “env.sample” file configuration
truffle migrate --network kovan --reset
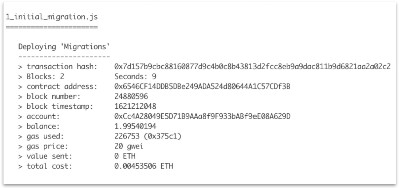
Helper scripts
Scripts to interact with deployed smart contract without any frontend implementation:
- fund-contract.js
npx truffle exec scripts/fund-contract.js --network kovan
- request-data.js
npx truffle exec scripts/request-data.js --network kovan
- read-contract.js
npx truffle exec scripts/read-contract.js --network kovan
● fund-contract.js
➢ Send 1 LINK to requesting contract
● request-data.js
➢ Chainlink request to be created from the requesting contract
● read-contract.js
➢ Read the data variable of the requesting contract (current price of pair ETH/USD)
Chainlink docs
Chainlink official website
Truffle Starter kit impl
Code a REAL WORLD dApp with Chainlink - Ethereum, Solidity, Web3.js
What Is Chainlink in 5 Minutes